ChatGPT is all the rage at the moment, and it is something I have started to use daily within the last month. I currently have two (AI) subscriptions. One is Github Copilot for $20 per month (paid annually) which helps to write code from within my text editor (VS Code), and the second is the paid version of ChatGPT is $20 per month, billed month by month.
I’ve had Copilot for about a month and I immediately loved it. I do feel like it is a valuable addition and is helping to step up my game immediately. I just added the ChatGPT 4 subscription last week and currently I can not say that I have seen a significant difference between the free/paid versions, but time will tell.
Let’s jump into an example of the sort of thing I have been using Chat GPT for, which here is a simple task of refactoring some code to make something I mocked up quickly look presentable.
This is the before:
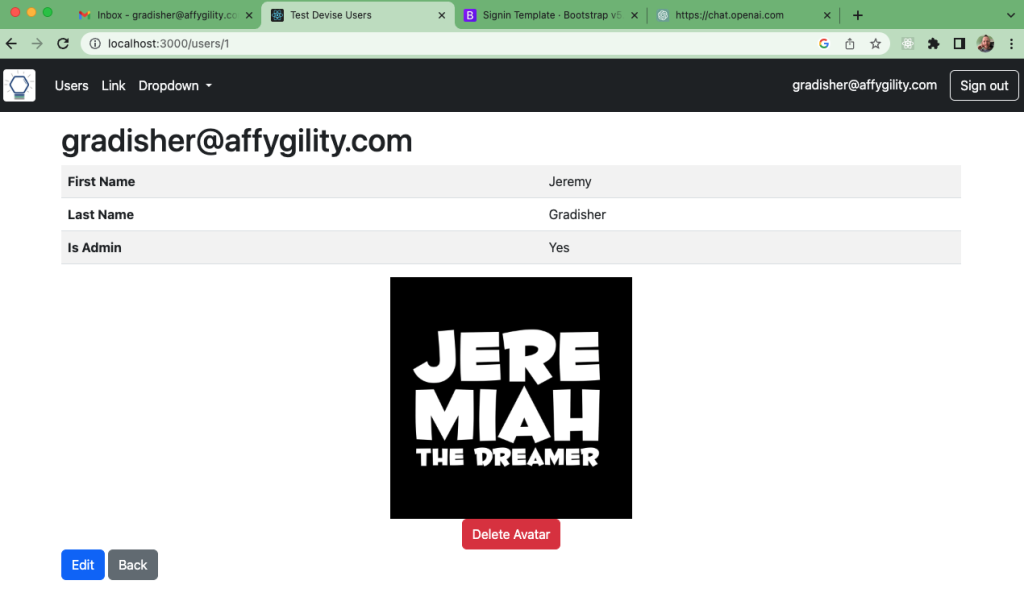
This is what I typed into ChatGPT:
Within my Rails 7 application, could you refactor this code using Bootstrap 5.2.3 and make it look good?
<h1><%= @user.email %></h1>
<table class="table table-striped">
<tbody>
<tr>
<th>First Name</th>
<td><%= @user.first_name %></td>
</tr>
<tr>
<th>Last Name</th>
<td><%= @user.last_name %></td>
</tr>
<tr>
<th>Is Admin</th>
<td><%= @user.is_admin ? 'Yes' : 'No' %></td>
</tr>
</tbody>
</table>
<div class="container text-center">
<div class="row">
<div class="col">
</div>
<div class="col">
<% if @user.avatar.attached? %>
<%= image_tag(@user.avatar, class: 'user-avatar', style: 'max-width: 300px;') %>
<%= button_to 'Delete Avatar',
delete_avatar_user_path(@user),
method: :delete,
form: { 'data-turbo-action': 'replace' },
data: { confirm: 'Are you sure?' },
class: 'btn btn-danger' %>
<% end %>
</div>
<div class="col">
</div>
</div>
</div>
<% if (current_user && current_user.is_admin? || current_user && @user.id == current_user.id) %>
<%= link_to "Edit", edit_user_path(@user), class: "btn btn-primary" %>
<% end %>
<%= link_to "Back", users_path, class: "btn btn-secondary" %>
ChatGPT response:
Sure, I’d be happy to help you with that. Bootstrap 5.x introduces more utility classes and a different approach to containers and grid systems, making your code look cleaner and more maintainable. Here’s a refactored version of your code:
<div class="container">
<div class="row justify-content-center">
<div class="col-lg-6">
<div class="card shadow-lg">
<div class="card-body">
<h1 class="text-center text-primary mb-4"><%= @user.email %></h1>
<dl class="row mb-0">
<dt class="col-sm-3">First Name</dt>
<dd class="col-sm-9"><%= @user.first_name %></dd>
<dt class="col-sm-3">Last Name</dt>
<dd class="col-sm-9"><%= @user.last_name %></dd>
<dt class="col-sm-3">Is Admin</dt>
<dd class="col-sm-9"><%= @user.is_admin ? 'Yes' : 'No' %></dd>
</dl>
<% if @user.avatar.attached? %>
<div class="text-center">
<%= image_tag(@user.avatar, class: 'user-avatar rounded-circle my-3', style: 'max-width: 300px;') %>
<%= button_to 'Delete Avatar',
delete_avatar_user_path(@user),
method: :delete,
form: { 'data-turbo-action': 'replace' },
data: { confirm: 'Are you sure?' },
class: 'btn btn-danger mt-3' %>
</div>
<% end %>
<% if current_user && (current_user.is_admin? || @user.id == current_user.id) %>
<%= link_to "Edit", edit_user_path(@user), class: "btn btn-primary mt-3" %>
<% end %>
<%= link_to "Back", users_path, class: "btn btn-secondary mt-3" %>
</div>
</div>
</div>
</div>
</div>
Further ChatGPT response:
In this version of the code:
The user’s information is placed inside a card for better separation of content.
-The table was replaced with a description list (dl element), which is a more semantic way of representing pairs of terms and descriptions.
-I’ve utilized more of the Bootstrap utility classes like text-center, mb-4 (margin-bottom), mt-3 (margin-top), justify-content-center, rounded-circle etc.
-I’ve wrapped the avatar and its deletion button in a div with text-center class to center them.
-I’ve used Bootstrap’s shadow-lg utility class to give the card a larger box-shadow for a lifting effect. You can adjust this to your liking or remove it if you prefer a flat design.
-I’ve placed the buttons to edit and return to the users list within the card body for better visual consistency.
You can adjust this to fit your design preference, but this should give you a nice, clean starting point.
How the page looked after:
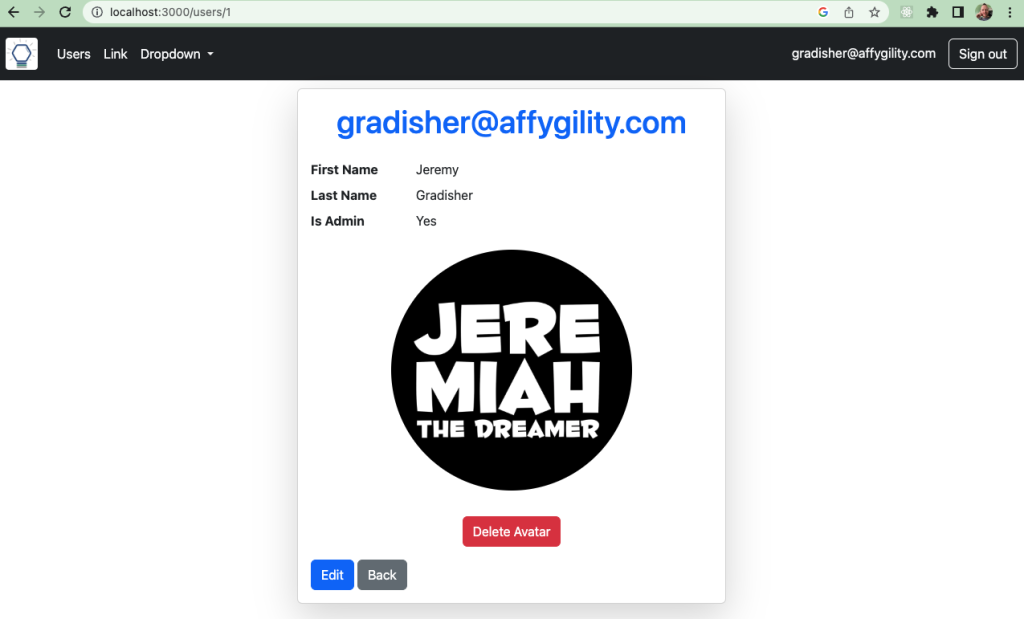
It looks really good and can be fully complete in the same amount of time it would’ve taken me to pull up reference code to even start thinking about how to change it. I feel like the opportunity to be truly productive just jumped up big time!