The Ahoy gem provides a solid foundation to track visits and events in Ruby, JavaScript, and native apps. Here’s how to add it to a Rails 7 application:
- Add the gem to your Gemfile:
Add the following line to your Gemfile:
gem 'ahoy_matey'
- Install the gem:
Run the following command in your terminal to install the gem:
bundle install
- Generate the Ahoy files:
After installing the gem, you need to generate some configuration and migration files. Run the following command in your terminal:
bin/rails generate ahoy:install
This will generate an initializer file at config/initializers/ahoy.rb and a migration file in db/migrate/ which creates two tables, ahoy_visits and ahoy_events.
- Run the migrations:
After you’ve generated the necessary files, you can apply the migrations with the following command:
bin/rails db:migrate
- Tracking visits and events:
Now that Ahoy is installed and set up, it will automatically track all visits to your application. If you want to track a specific event, you can use the ahoy.track method in your controllers, views, or models. Here’s an example:
ahoy.track "Viewed book", title: "Hotwire"
- Tracking in JavaScript:
Ahoy can also track events in JavaScript. First, you need to add the ahoy.js file to your app/javascript/packs/application.js:
import "ahoy.js";
Then you can use the ahoy.track function in your JavaScript code like this:
ahoy.track("Viewed book", { title: "Hotwire" });
The ahoy.track function takes two arguments: the name of the event, and an object containing any properties you want to associate with the event.
That’s it! You’ve now installed and set up the Ahoy gem in your Rails 7 application.
Remember to check out the Ahoy documentation for more details on how to use the gem, as there are many other features and options available.
Testing if Ahoy is tracking
Testing if Ahoy is correctly tracking visits and events can be done by checking your database and application logs. You could also write automated tests to confirm that Ahoy is functioning as expected. Here’s how to do these:
- Manual testing via development logs:
In the Rails development environment, you can check the Rails server logs in the console to see if Ahoy is tracking visits and events correctly.
For example, if you have a line like ahoy.track “Viewed book”, title: “Hotwire” in one of your actions and you perform that action, you should see an SQL INSERT statement in your logs indicating that a new event has been recorded.
The log line might look something like this:
(0.1ms) BEGIN
Ahoy::Event Create (0.4ms) INSERT INTO "ahoy_events"...
(0.1ms) COMMIT
- Checking the database directly:
You can check the ahoy_visits and ahoy_events tables in your database to see if visits and events are being tracked.
Here’s how you might check the ahoy_events table in Rails console:
rails console
Then in the Rails console, run:
Ahoy::Event.all
This will give you a list of all the events that Ahoy has tracked. You can inspect these records to see if they match your expectations.
- Writing automated tests:
If you want to write automated tests to ensure that Ahoy is tracking events correctly, you could write a test that performs an action, and then checks that an Ahoy::Event record was created with the expected properties.
Here’s an example using RSpec and FactoryBot:
require 'rails_helper'
RSpec.describe BooksController, type: :controller do
describe 'GET #show' do
let(:book) { create(:book, title: 'Hotwire') }
it 'tracks viewed book event' do
expect do
get :show, params: { id: book.id }
end.to change(Ahoy::Event, :count).by(1)
event = Ahoy::Event.last
expect(event.name).to eq('Viewed book')
expect(event.properties).to eq('title' => 'Hotwire')
end
end
end
In this test, we are simulating a GET request to the show action of the BooksController. We then expect that this results in an Ahoy::Event record being created, and we check that the name and properties of the event are as expected.
Remember to adapt these methods to fit your particular application and setup.
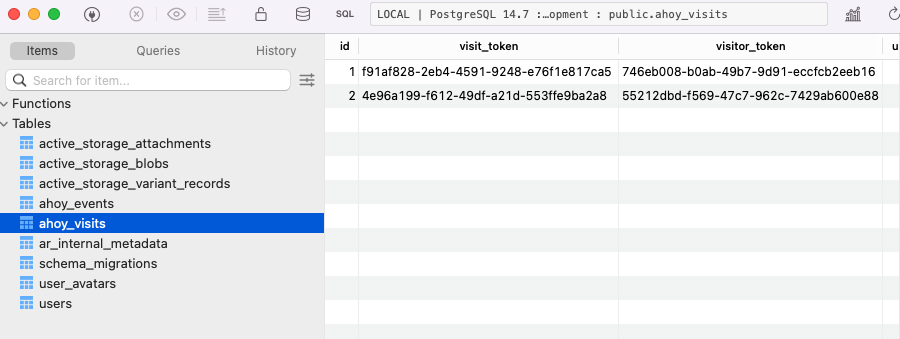
You would add an event of visiting the users#index page by invoking the ahoy.track method in your UsersController#index action. Here’s how you can do it:
class UsersController < ApplicationController
def index
@users = User.all
ahoy.track "Visited Users Index"
end
end
In this example, every time the index action is called, Ahoy will create a new event named “Visited Users Index”. You can view these events in your ahoy_events table.
If you want to pass additional properties with the event, you can pass a second argument to ahoy.track which is a hash of the properties you want to record. For example:
class UsersController < ApplicationController
def index
@users = User.all
ahoy.track "Visited Users Index", { "Total Users": @users.count }
end
end
In this case, Ahoy will record an event each time the index action is called, and the event will have a property Total Users that records the total number of users at the time of the visit.
Remember that the ahoy.track method can be called from any controller action, view, or model, so you can use it to record a wide variety of different events in your application.
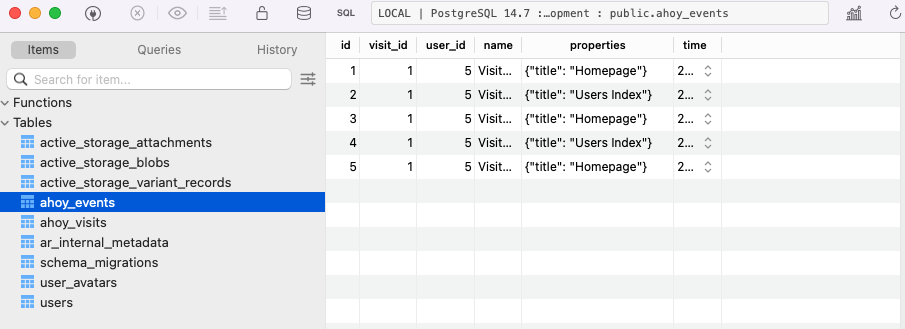